I have been working on the img-genner library(repo here) for quite a long time now, over three years if my memory serves me correctly. It contains a number of different modules, and frankly, it is a bit of a Frankenstein. At first it was about polygon graphics, then it became about pixel ‘glitch’ effects, such as pixel sorting and other stuff, partially because I wanted to write the algorithms myself and gain an understanding of them from implementation.
I’m still not quite sure about the triangularization algorithm(which we won’t demonstrate here because I’m not sure it still works), but for the most part it has worked in that way.
For the examples here I am assuming that you have a functional roswell/common lisp environment with quicklisp and all the other amenities.
Getting the library
First clone it to your local projects directory, the command that will work for you will vary from this if you are not using roswell. As of now I have no plans to put this library on quicklisp unless there is some interest.
$> git clone https://github.com/epsilon-phase/img-genner ~/.roswell/local-projects/img-genner
Now you should be able to execute ros run
and successfully run (ql:quickload :img-genner)
in the repl. But that’s probably not a great experience. We need an environment that is at least somewhat durable and reset-able. So let’s make and go into a project folder
$> mkdir post-examples-that-are-totally-cool $> cd post-examples-that-are-totally-cool
Add two very cool images.
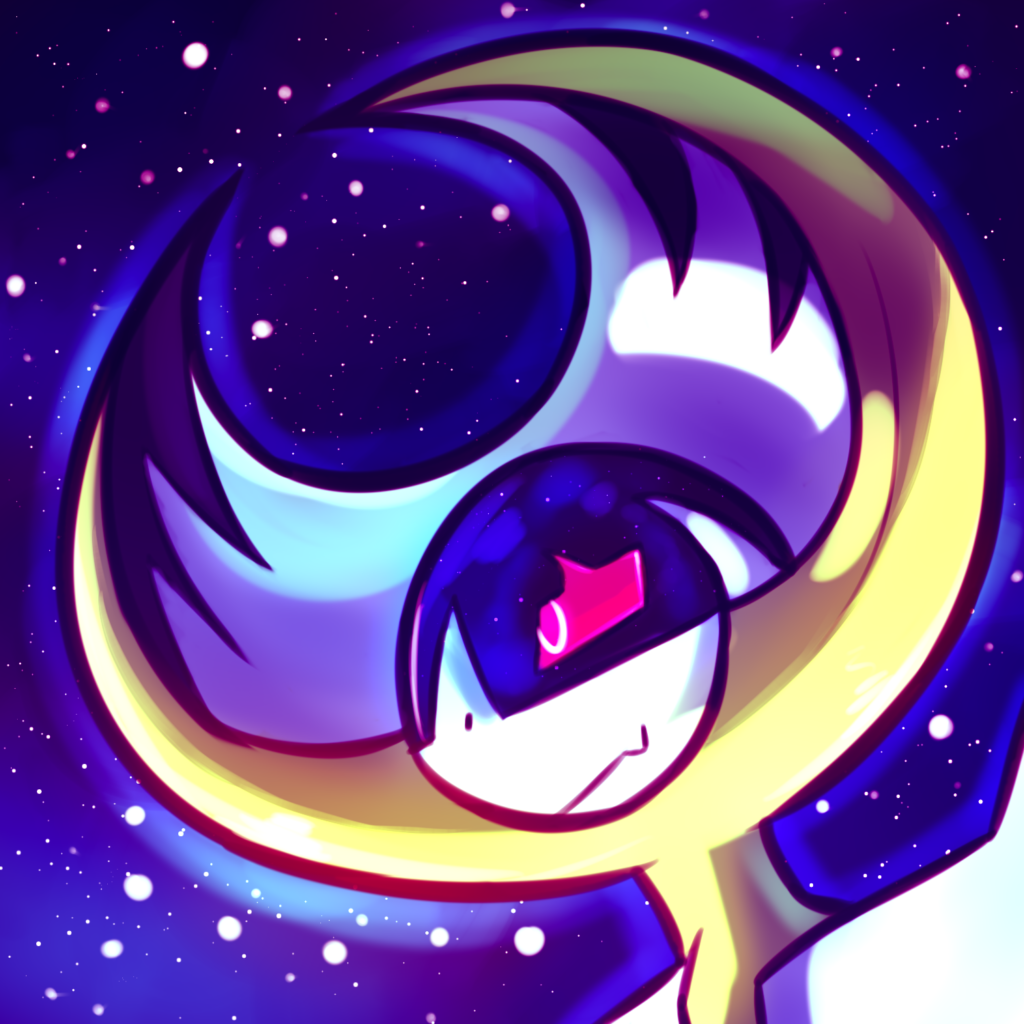
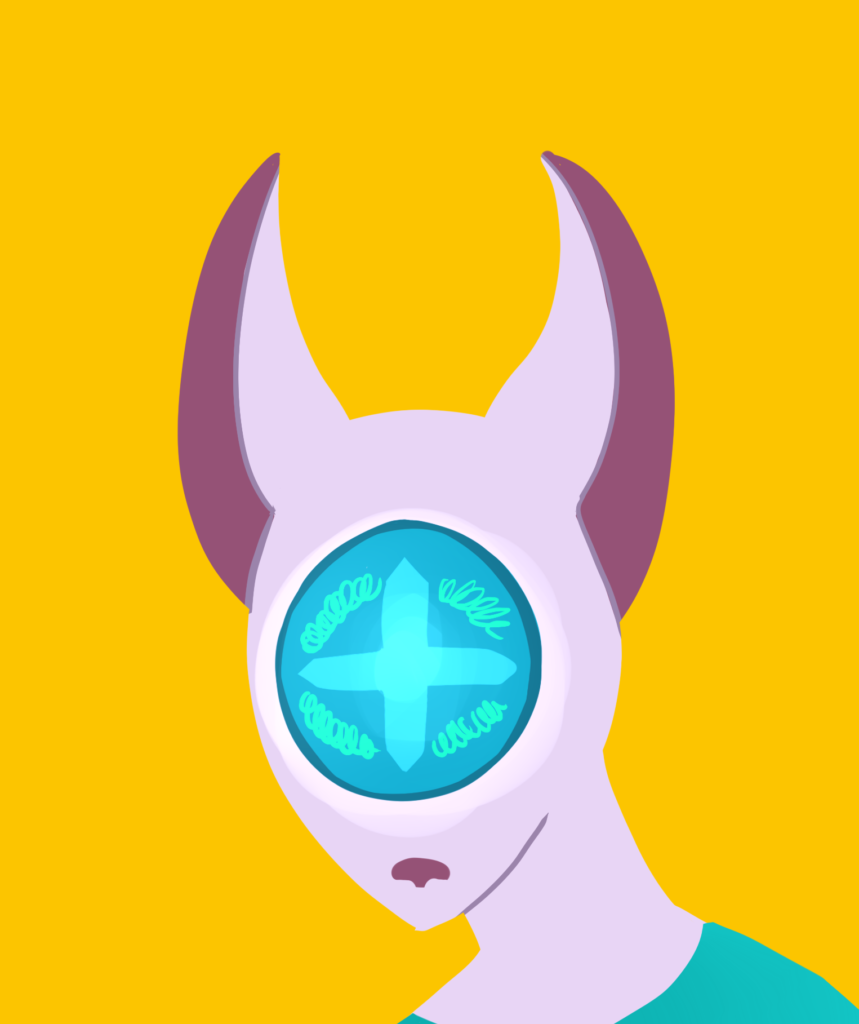
And a file called something like post.lisp
To start we should probably add load the library, but it would also be useful to have some way to clean up the folder if we don’t like what we have so far.
(ql:quickload :img-genner) (defun clean() (loop for i in (directory "*.png") when(not (member (pathname-name i) '("lunala" "fox-profile") :test #'string=)) do(print i) and do(delete-file i)))
With that we can clear any new images that we decide to make.
We should also add (ql:quickload :img-genner)
to the top of the file to make loading it easier(no need for defpackage or asdf yet).
So, let’s see, let’s… downscale the lunala picture. So add this to the end of post.lisp
; Using defparameter reloads the file each time the compiler ; processes it again, which might be useful (defparameter *lunala* (img-genner:load-image "lunala.png")) (img-genner:save-image (img-genner:downscale-x2 (img-genner:downscale-x2 *lunala*)) "lunala-smol.png")
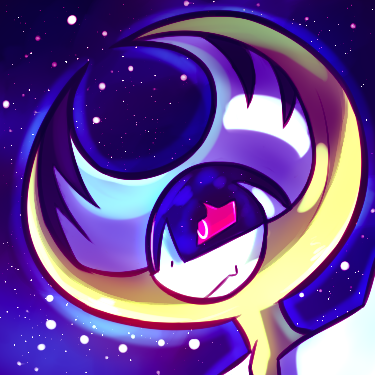
Well, that looks more or less as you would expect.
Let’s also load the fox-profile picture and then combine the two images.
(defparameter *fox-profile* (img-genner:load-image "fox-profile.png")) ; The 30 30 refers to the size of the tiles in pixels. ; In general, the smaller the tiles the longer this takes (img-genner:save-image (img-genner:mosaify *lunala* *fox-profile* 30 30) "mosaic.png")
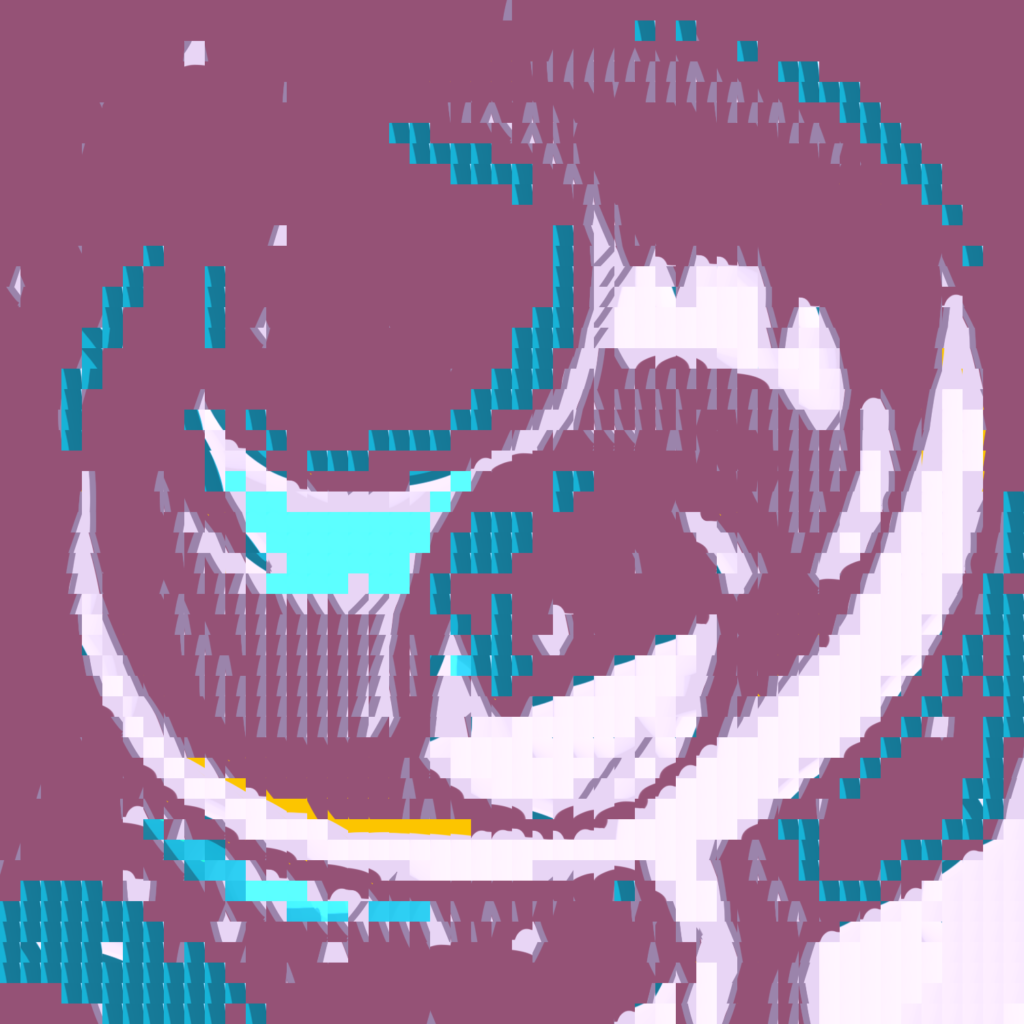
So this has combined the two images by replacing blocks with the most similar blocks of the other image. We can change the way that it scores similarity by changing the distance metric used.
If we use the color-brightness
distance function then we can care more about the brightness of the tiles rather than color.
(img-genner:save-image (img-genner:mosaify *lunala* *fox-profile* 30 30 :distance #'img-genner:color-brightness) "mosaic-bright.png")
You can see the result below.
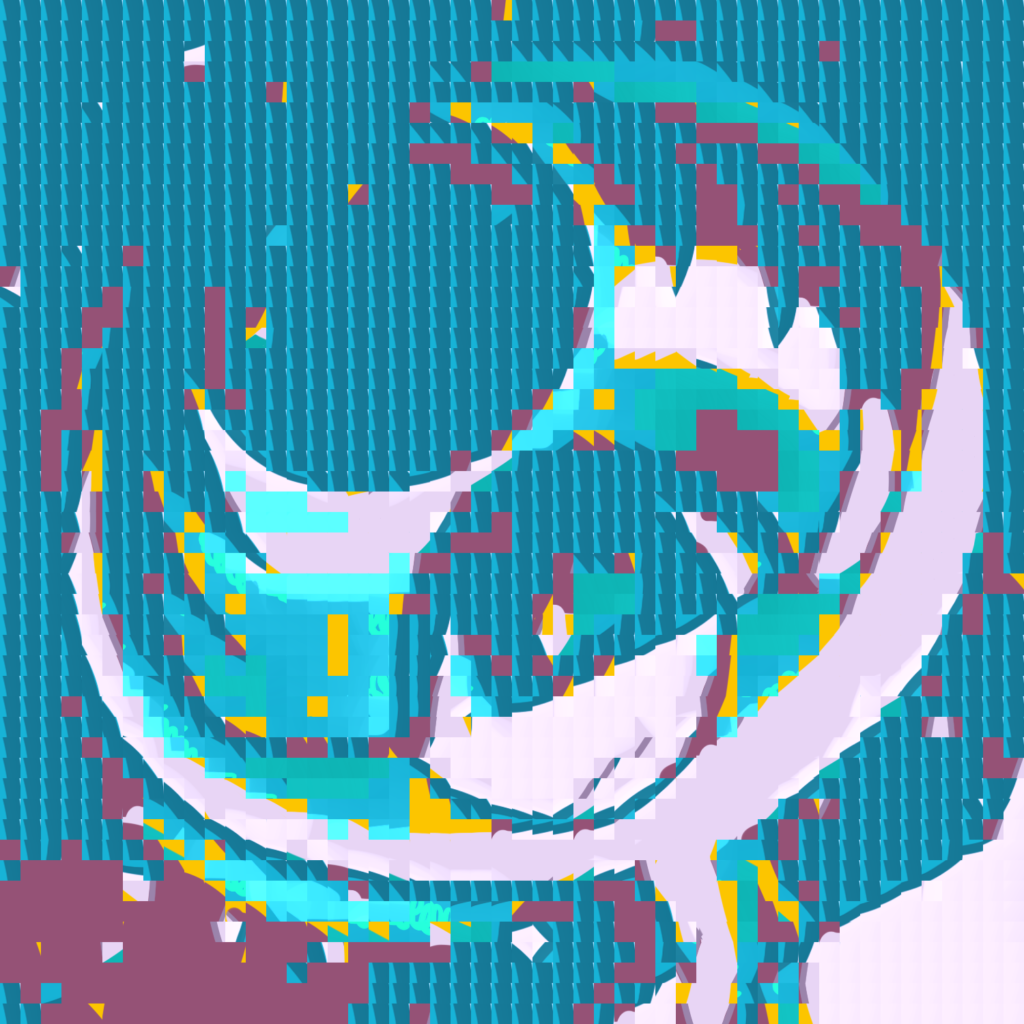
And before I finish this post, let me show you a pixel-sort from the library. (This will be rather slow)
(img-genner:save-image (img-genner:fuck-it-up-pixel-sort *lunala* 600 600) "sorted.png")
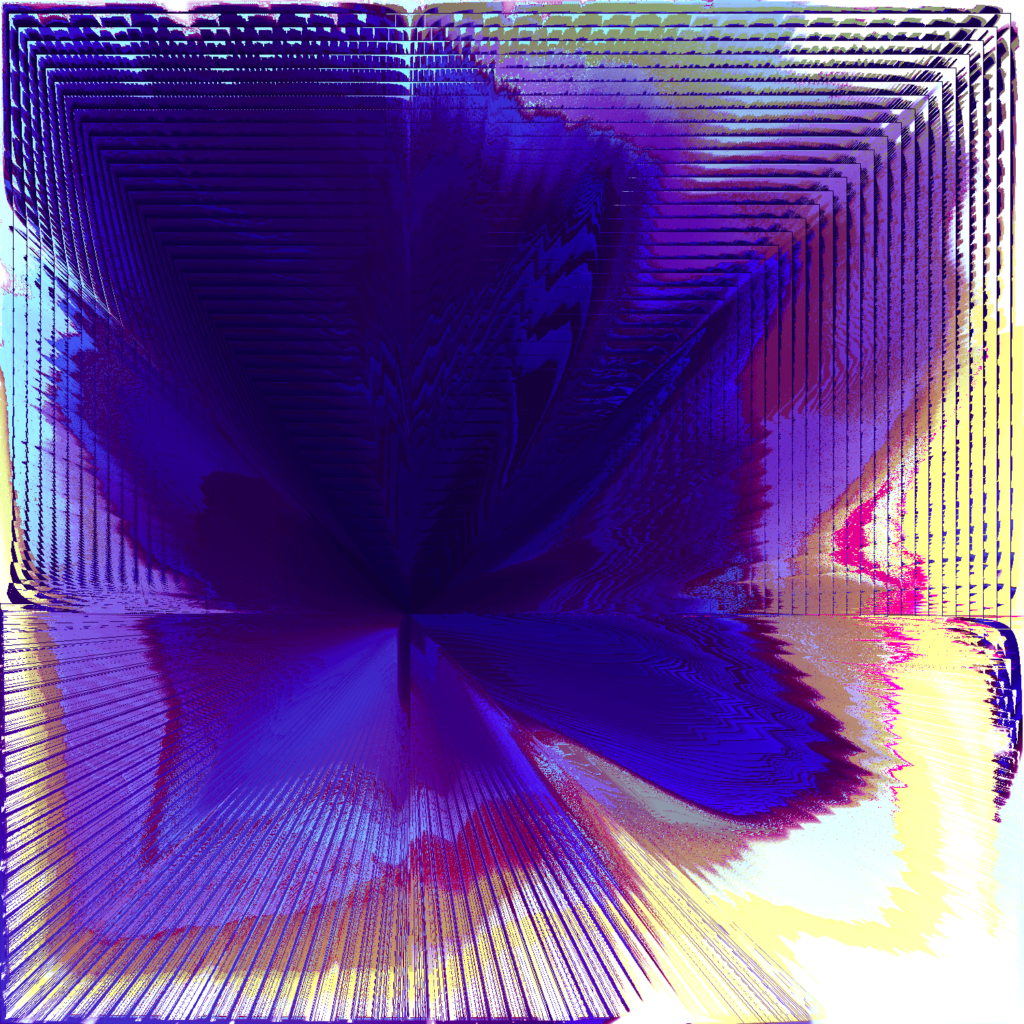
Leave a Reply
Only people in my network can comment.